Python
Python: list clusters, hosts and VMs
Just following on from my previous article (HERE) on using Python Pyvmomi SDK wrapper for the vSphere Web Services API this time I show you how to iterate through the Web Services API tree. In numerous posts and VMUG sessions, I have given dating back to 2007 I have discussed that the objects in vSphere Web API are laid out in a hierarchical tree. Probably using the same relationship that objects have inside the vCenter Database. Here is the current diagram form the Vmware documentation. At the root of the tree, there is a folder; it contains the datacenter object. Underneath the Datacenter object is several other folders containing related objects. One for hosts, one for VMs, one for Networks and one for Storage.

The objective of this posts Python script is to move down the left-hand side of the tree list each object and finally getting to a list of VMs. The script below assumes you have to use the setup for authenticating to vSphere as in the previous script (HERE) and only contains the difference of what we are trying to achieve this time.
content = si.RetrieveContent()
# now loop through any object that is tagged as "rooFolder"
for child in content.rootFolder.childEntity:
if hasattr(child, 'hostFolder'):
datacenter = child # first object will be a datacentre
hostFolder = datacenter.hostFolder # now assign the datacenter to a hostfolder variable
cluster = hostFolder.childEntity # childs of hosts folders are clusters
print("Datacenter name: " + child.name)
# now loop through the clusters object
for clusterList in cluster:
print(clusterList.name)
if hasattr(clusterList, 'host'): #if a child is tagged is host loop through the child
for host in clusterList.host:
print(host.name)
for vm in host.vm: # host.vm holds a list of vm associated to that host
print(" VM name: " + vm.name)
You can see from this code that we first find the folder tagged as rootfolder. This contains the datacenter object. Which in turn contains a hostfolder object which in turn contains a computer resource folder (the cluster). Which in turn contains the Host objects. This is where we stop. But hold on arent’t we suppose to list the VMs too? Well the VM objects are actually in a different folder. We observed that last time. However each host as a parameter called host.vm which is a list just of the name of each VM that is associated with that particular host.
Next time we will see how we can build to lists of objects and compare them so we cannot only list up the the VMs but list there properties too.
Python and VMware SDK
So I’m making a big push to learn Python. In IT don’t think you can survive without it. For those who don’t know me, I’ve never been a professional programmer. I learned enough C#.net 13 years ago to be dangerous. I did it so I could learn how to automate VMware by building my own tools. Thirteen years later and a plethora of tools later including vDisk Informer, vSphere Plugin Wizard and vRealize Automation icon changer I’m looking at starting again. This time with python. The first job as last time, the VMware programmer’s equivalent of “Hello World” which is to list the VMs on your vSphere environment. To make my life easier, I decided upon the Python SDK wrapper pyvmomi . So here’s how to do it in my favourite python IDE PyCharm.
Step pip install pyvmomi using the below command: pip install –upgrade pyvmomi

Now fire up Pycharm and create a new project.

Now the next step caught me out as a novice. It’s not enough to import the pyvmomi library in your code, you also have to add it through pycharm in your project.
Click on File > Settings >

Now expand your Project and click on “Project Interrupter”

Click on the + symbol on the right

Now in the search box search for pyvmomi and select it.

Now click on Install Package

Once it’s finished installing it should look like this:

Now underneath your project, create a new python file and let’s get coding.
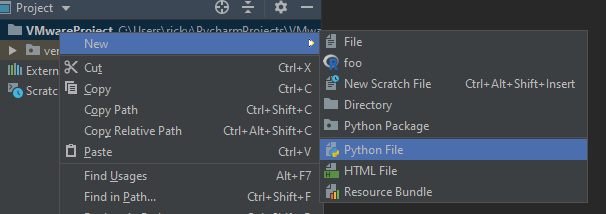
Cut and paste the following code into your newly created python file. Make sure you change the hostname, port, username and password to reflect your environment. I have added comments to explain how to code works inline with the code.
# first import the sdk wrapper library
from pyVim.connect import SmartConnect, Disconnect
from pyVmomi import vim
# import the ssl library to handle http
import ssl
# now setup your connection properties
host = "vcsa6.demo.local"
port = 443
user = "administrator@vsphere.local"
password = "PassW0rd123"
# now connect to your vCenter/vSphere
context = None
if hasattr(ssl, '_create_unverified_context'):
context = ssl._create_unverified_context()
si = SmartConnect(host=host,
user=user,
pwd=password,
port=port,
sslContext=context)
if not si:
print("Could not connect to the specified host using specified "
"username and password")
# no grab the data model of the SDK tree
content = si.RetrieveContent()
# now loop through any object that is tagged as "vmFolder"
for child in content.rootFolder.childEntity:
if hasattr(child, 'vmFolder'):
datacenter = child
vmFolder = datacenter.vmFolder
vmList = vmFolder.childEntity
# now grab the child nodes of any vmFolder object
for vm in vmList:
if hasattr(vm, 'childEntity'):
vmList2 = vm.childEntity
# now loop through the child ojbects printing their user friendly name
for c in vmList2:
vmproperties = c.summary
print(vmproperties.config.name)
It can be difficult to visualize what is going on since you don’t have a picture of the data model. I learned this many years ago and to give you help, you should watch my video on automating VMware using the REST API. Pyvmomi is not using the REST API, but my video also gives some clue to how the data model is laid out. You find it useful. Click HERE to watch. Remember MoB is your friend!